일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
- Kotlin
- 앱
- Compose
- 테스트
- binding
- textview
- Navigation
- activity
- viewmodel
- Dialog
- ScrollView
- appbar
- 안드로이드
- Button
- CustomScrollView
- tabbar
- scroll
- Coroutines
- TEST
- LifeCycle
- drift
- intent
- android
- textfield
- DART
- 계측
- 앱바
- livedata
- Flutter
- data
- Today
- Total
Study Record
[Flutter] Dialog (다이얼로그), 모서리가 둥근 다이얼로그 본문
🎁 Dialog
플러터에서 기본적인 다이얼로그는 showDialog 함수를 사용하여 Dialog 위젯을 띄운다.
showDialog(
context: context,
builder: (BuildContext context) {
return Dialog(
alignment: Alignment.center,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(16.0)),
),
child: Container(...),
);
},
);
Dialog 위젯의 child 로 다이얼로그의 화면을 직접 그릴 수 있고 alignment 인자로 다이얼로그의 화면에서의 위치를 정할 수 있다.
😶 간단한 예시)
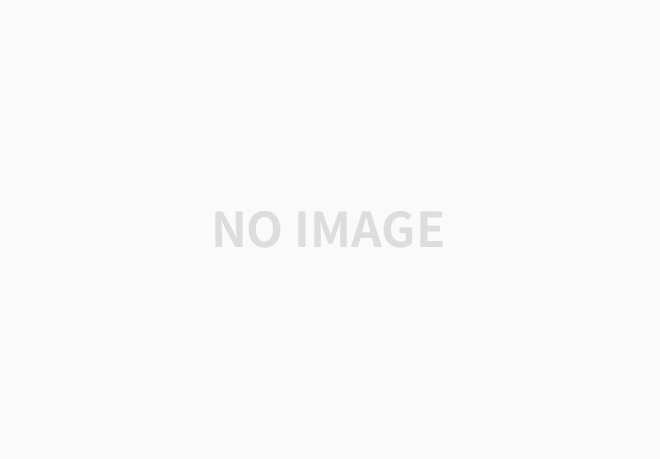
import 'package:flutter/material.dart';
void main() => runApp(MaterialApp(
home: MyApp(),
debugShowCheckedModeBanner: false,
));
class MyApp extends StatelessWidget {
const MyApp({Key? key}) : super(key: key);
@override
Widget build(BuildContext context) {
return Scaffold(
body: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
mainAxisAlignment: MainAxisAlignment.center,
children: [
TextButton(
onPressed: () {
startDialog(context);
},
child: Text("show Dialog"),
),
],
),
);
}
void startDialog(BuildContext context) {
showDialog(
context: context,
builder: (BuildContext context) {
return Dialog(
alignment: Alignment.center,
child: Container(
color: Colors.deepOrangeAccent[100],
padding: EdgeInsets.all(16.0),
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Text("Dialog Test"),
const SizedBox(height: 16.0),
ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("확인"),
),
],
),
),
);
},
);
}
}
😶 모서리가 둥근 다이얼로그
Dialog 위젯의 shape 를 모서리가 둥근 경계선을 나타내는 RoundedRectangleBorder 로 하고 child 위젯의 최상단 위젯을 shape 와 마찬가지로 모서리가 둥근 모양으로 설정해 주면 된다.
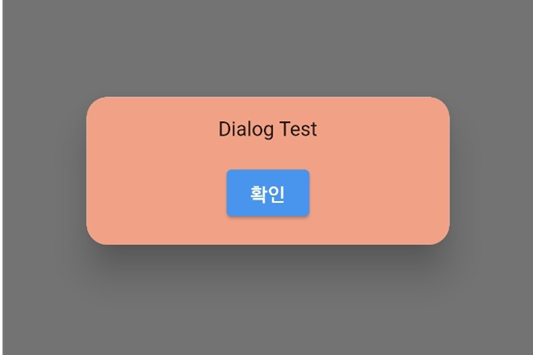
import 'package:flutter/material.dart';
Dialog(
alignment: Alignment.center,
shape: RoundedRectangleBorder(
borderRadius: BorderRadius.all(Radius.circular(16.0)),
),
child: Container(
decoration: BoxDecoration(
color: Colors.deepOrangeAccent[100],
borderRadius: BorderRadius.all(Radius.circular(16.0)),
),
padding: EdgeInsets.all(16.0),
child: Column(
mainAxisSize: MainAxisSize.min,
mainAxisAlignment: MainAxisAlignment.spaceEvenly,
children: [
Text("Dialog Test"),
const SizedBox(height: 16.0),
ElevatedButton(
onPressed: () {
Navigator.of(context).pop();
},
child: Text("확인"),
),
],
),
),
);
😶 Elevation
Dialog() 의 elevation 인자로 elevation 값을 조절할 수 있다.
showDialog(
context: context,
builder: (BuildContext context) {
return Dialog(
elevation: 0.0,
...
);
}
);
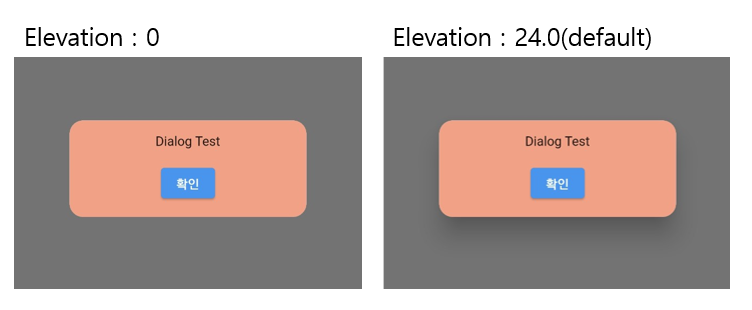
😶 다이얼로그 종료
showDialog() 함수의 barrierDismissible 을 false 로 하면 다이얼로그 밖을 클릭했을 때 다이얼로그가 종료되지 않는다. true 이면 다이얼로그 밖을 클랙 했을 때 다이얼로그가 종료된다.
showDialog(
barrierDismissible: false,
context: context,
builder: (BuildContext context) {
return Dialog( ... );
}
);
Dialog class - material library - Dart API
A Material Design dialog. This dialog widget does not have any opinion about the contents of the dialog. Rather than using this widget directly, consider using AlertDialog or SimpleDialog, which implement specific kinds of Material Design dialogs. link To
api.flutter.dev
'Flutter' 카테고리의 다른 글
[Flutter] 뒤로 가기(back press) 버튼 컨트롤 (WillPopScope) (0) | 2023.04.24 |
---|---|
[Flutter] 앱 이름 바꾸기 (app name) (0) | 2023.04.22 |
[AndroidStudio] 자동 완성 설정하기 (Live Templates) (0) | 2023.04.14 |
[Flutter] Run start ms-settings:developers (0) | 2023.04.07 |
[Flutter] 입력 폼이 포함된 화면 자연스럽게 구성하기 (0) | 2023.04.07 |